Simple Garbage Collector Program In Javarubackup
Interface Overview | Tutorial Slides | FAQ | Example | Download | License |
GC (Garbage collection) is the process by which JVM cleans objects (unused objects) from heap to reclaim heap space in java. What is Automatic Garbage Collection in JVM heap memory in java? The page on the D language's website about garbage collection - which is natively compiled and has an optional garbage collector - also seems to hint that some background program runs alongside the original executable program to implement garbage collection. D is a systems programming language with support for garbage collection. Java Garbage Collection In java, garbage means unreferenced objects. Garbage Collection is process of reclaiming the runtime unused memory automatically. In other words, it is a way to destroy the unused objects.
http://www.hpl.hp.com/personal/Hans_Boehm/gc,and before that athttp://reality.sgi.com/boehm/gc.htmland before that atftp://parcftp.xerox.com/pub/gc/gc.html. ]The Boehm-Demers-Weiserconservative garbage collector canbe used as a garbage collectingreplacement for C malloc or C++ new.It allows you to allocate memory basically as you normally would,without explicitly deallocating memory that is no longer useful.The collector automatically recycles memory when it determinesthat it can no longer be otherwise accessed.A simple example of such a use is givenhere.
The collector is also used by a number of programming languageimplementations that either use C as intermediate code, wantto facilitate easier interoperation with C libraries, orjust prefer the simple collector interface.For a more detailed description of the interface, seehere.
Alternatively, the garbage collector may be used asa leak detectorfor C or C++ programs, though that is not its primary goal.
The arguments for and against conservative garbage collectionin C and C++ are brieflydiscussed inissues.html. The beginnings ofa frequently-asked-questions list are here.
Empirically, this collector works with most unmodified C programs,simply by replacingmalloc with GC_malloc calls,replacing realloc with GC_realloc calls, and removingfree calls. Exceptions are discussedin issues.html.
Where to get the collector
Many versions are available in thegc_source subdirectory.Currently a fairly recent stable version isgc-8.0.4.tar.gz.Note that this uses the new version numbering schemeand may require a separate libatomic_ops download (see below).
If that fails, try the latest explicitly numbered versionin gc_source.Later versions may contain additional features, platform support,or bug fixes, but are likely to be less well tested.
Version 7.3 and later require that you download a correspondingversion of libatomic_ops, which should be availablein https://github.com/ivmai/libatomic_ops/wiki/Download.The current version is also available asgc_source/libatomic_ops-7.6.10.tar.gz.You will need to place that in a libatomic_opssubdirectory.
Previously it was best to use corresponding versions of gcand libatomic_ops, but for recent versions, it should be OK tomix and match them.
Starting with 8.0, libatomic_ops is only required if the compiler doesnot understand C atomics.
The development version of the GC source code now resides on github,along with the downloadable packages.The GC tree itself is athttps://github.com/ivmai/bdwgc/.The libatomic_ops tree required by the GC is athttps://github.com/ivmai/libatomic_ops/.
To build a working version of the collector, you will need to dosomething like the following, where D is the absolutepath to an installation directory:This will require that you have C and C++ toolchains, git,automake, autoconf, and libtool alreadyinstalled.
Historical versions of the source can still be foundon the SourceForge site (project 'bdwgc')here.
The garbage collector code is copyrighted byHans-J. Boehm,Alan J. Demers,Xerox Corporation,Silicon Graphics,andHewlett-Packard Company.It may be used and copied without payment of a fee under minimal restrictions.See the README file in the distribution or thelicense for more details.IT IS PROVIDED AS IS,WITH ABSOLUTELY NO WARRANTY EXPRESSED OR IMPLIED. ANY USE IS AT YOUR OWN RISK.
Platforms
The collector is not completely portable, but the distributionincludes ports to most standard PC and UNIX/Linux platforms.The collector should work on Linux, *BSD, recent Windows versions,MacOS X, HP/UX, Solaris,Tru64, Irix and a few other operating systems.Some ports are more polished than others.There are instructions for porting the collectorto a new platform.Irix pthreads, Linux threads, Win32 threads, Solaris threads(old style and pthreads),HP/UX 11 pthreads, Tru64 pthreads, and MacOS X threads are supportedin recent versions.
Separately distributed ports
For MacOS 9/Classic use, Patrick Beard's latest port were once available fromhttp://homepage.mac.com/pcbeard/gc/.Several Linux and BSD versions provide prepacked versions of the collector.The Debian port can be found athttps://packages.debian.org/sid/libgc-dev.
Scalable multiprocessor versions
Kenjiro Taura, Toshio Endo, and Akinori Yonezawa developed a parallel collectorbased on this one. It was once available from http://www.yl.is.s.u-tokyo.ac.jp/gc/Their collector takes advantage of multiple processorsduring a collection. Starting with collector version 6.0alpha1we also do this, though with more modest processor scalability goals.Our approach is discussed briefly inscale.html.Some Collector Details
The collector uses a mark-sweep algorithm.It provides incremental and generationalcollection under operating systems which provide the right kind ofvirtual memory support. (Currently this includes SunOS[45], IRIX,OSF/1, Linux, and Windows, with varying restrictions.)It allows finalization codeto be invoked when an object is collected.It can take advantage of type information to locate pointers if suchinformation is provided, but it is usually used without such information.ee the README andgc.h files in the distribution for more details.For an overview of the implementation, see here.
The garbage collector distribution includes a C string(cord) package that providesfor fast concatenation and substring operations on long strings.A simple curses- and win32-based editor that represents the entire fileas a cord is included as asample application.
Performance of the nonincremental collector is typically competitivewith malloc/free implementations. Both space and time overhead arelikely to be only slightly higherfor programs written for malloc/free(see Detlefs, Dosser and Zorn'sMemory Allocation Costs in Large C and C++ Programs.)For programs allocating primarily very small objects, the collectormay be faster; for programs allocating primarily large objects it willbe slower. If the collector is used in a multithreaded environmentand configured for thread-local allocation, it may in some casessignificantly outperform malloc/free allocation in time.
We also expect that in many cases any additional overheadwill be more than compensated for by decreased copying etc.if programs are writtenand tuned for garbage collection.The beginnings of a frequently asked questions list for thiscollector are here.
The following provide information on garbage collection in general:
Paul Wilson's garbage collection ftp archive and GC survey.
The Ravenbrook Memory Management Reference.
David Chase'sGC FAQ.
Richard Jones'GC page andhis book.
The following papers describe the collector algorithms we useand the underlying design decisions ata higher level.
(Some of the lower level details can be foundhere.)
The first one is not availableelectronically due to copyright considerations. Most of the others aresubject to ACM copyright.
Boehm, H., 'Dynamic Memory Allocation and Garbage Collection', Computers in Physics9, 3, May/June 1995, pp. 297-303. This is directed at an otherwise sophisticatedaudience unfamiliar with memory allocation issues. The algorithmic details differfrom those in the implementation. There is a related letter to the editor and a minorcorrection in the next issue.
Boehm, H., and M. Weiser,'Garbage Collection in an Uncooperative Environment',Software Practice & Experience, September 1988, pp. 807-820.
Boehm, H., A. Demers, and S. Shenker, 'Mostly Parallel Garbage Collection', Proceedingsof the ACM SIGPLAN '91 Conference on Programming Language Design and Implementation,SIGPLAN Notices 26, 6 (June 1991), pp. 157-164.
Boehm, H., 'Space Efficient Conservative Garbage Collection', Proceedings of the ACMSIGPLAN '93 Conference on Programming Language Design and Implementation, SIGPLANNotices 28, 6 (June 1993), pp. 197-206.
Boehm, H., 'Reducing Garbage Collector Cache Misses', Proceedings of the 2000 International Symposium on Memory Management .Official version.Technical report version. Describes the prefetch strategyincorporated into the collector for some platforms. Explains whythe sweep phase of a 'mark-sweep' collector should not really bea distinct phase.
M. Serrano, H. Boehm,'Understanding Memory Allocation of Scheme Programs',Proceedings of the Fifth ACM SIGPLAN International Conference onFunctional Programming, 2000, Montreal, Canada, pp. 245-256.Official version.Earlier Technical Report version. Includes some discussion of thecollector debugging facilities for identifying causes of memory retention.
Boehm, H.,'Fast Multiprocessor Memory Allocation and Garbage Collection',HP Labs Technical Report HPL 2000-165. Discusses the parallelcollection algorithms, and presents some performance results.
Boehm, H., 'Bounding Space Usage of Conservative Garbage Collectors',Proceeedings of the 2002 ACM SIGPLAN-SIGACT Symposium on Principles ofProgramming Languages, Jan. 2002, pp. 93-100.Official version.Technical report version.Includes a discussion of a collector facility to much more reliably test forthe potential of unbounded heap growth.
The following papers discuss language and compiler restrictions necessary to guaranteedsafety of conservative garbage collection.
We thank John Levine and JCLT for allowingus to make the second paper available electronically, and providing PostScript for the finalversion.
Boehm, H., ``SimpleGarbage-Collector-Safety', Proceedingsof the ACM SIGPLAN '96 Conference on Programming Language Designand Implementation.
Boehm, H., and D. Chase, ``A Proposal for Garbage-Collector-Safe C Compilation',Journal of C Language Translation 4, 2 (Decemeber 1992), pp. 126-141.
Other related information:
The Detlefs, Dosser and Zorn's Memory Allocation Costs in Large C and C++ Programs. This is a performance comparison of the Boehm-Demers-Weiser collector to malloc/free,using programs written for malloc/free.
Joel Bartlett's mostly copying conservative garbage collector for C++.
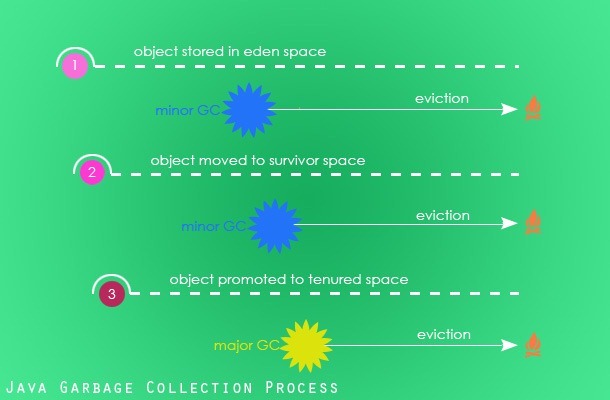
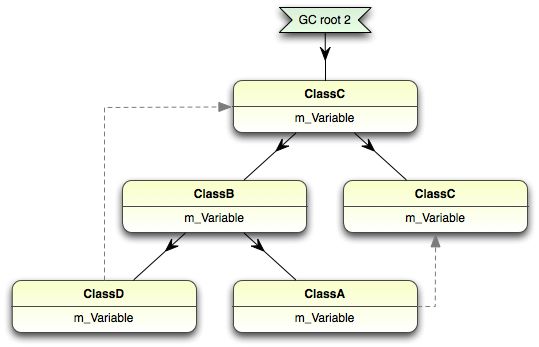
John Ellis and David Detlef's Safe Efficient Garbage Collection for C++ proposal.
Default Garbage Collector Java 8
Henry Baker's paper collection.
Slides for Hans Boehm's Allocation and GC Myths talk.
This section has not been updated recently.
Known current users of some variant of this collector include:
The runtime system for GCJ,the static GNU java compiler.
W3m, a text-based web browser.
Some versions of the Xerox DocuPrint printer software.
The Mozilla project, as leakdetector.
The Mono project,an open source implementation of the .NET development framework.
The DotGNU Portable.NETproject, another open source .NET implementation.
The Irssi IRC client.

The Berkeley Titanium project.
The NAGWare f90 Fortran 90 compiler.

Elwood Corporation's Eclipse Common Lisp system, C library, and translator.
The BiglooSchemeand Camloo MLcompilerswritten by Manuel Serrano and others.
Java Run Garbage Collector
Brent Benson's libscheme.
Garbage Collector In Java
The MzScheme scheme implementation.
The University of Washington Cecil Implementation.
The Berkeley Sather implementation.
The Berkeley Harmonia Project.
The Toba Java VirtualMachine to C translator.
The Gwydion Dylan compiler.
The GNU Objective C runtime.
Macaulay 2, a system to supportresearch in algebraic geometry and commutative algebra.
The Vesta configuration managementsystem.
Visual Prolog 6.
Asymptote LaTeX-compatiblevector graphics language.A simple illustration of how to build anduse the collector..
Paper corresponding to above slide set.(Technical Report version.)
For current updates on the collector and information on reporting issues,please see the bdwgc github page.
There used to be a pair of mailing lists for GC discussions. Those are no longer active.Please see the above page for pointers to the archives.
Some now ancient discussion of the collector took place on the gccjava mailing list, whose archives appearhere, and also ongclist@iecc.com.
Comments and bug reports may also be sent to(boehm@acm.org), but reporting issues ongithub is strongly preferred.
- Related Questions & Answers
- Selected Reading
Java Garbage collector tracks the live object and objects which are no more need are marked for garbage collection. It relieves developers to think of memory allocation/deallocation issues.
JVM uses the heap, for dynamic allocation. In most of the cases, the operating systems allocate the heap in advance which is then to be managed by the JVM while the program is running. It helps in following ways −
Faster object creation as Operating system level synchronization is no more needed for each object. Object Allocation takes some memory and increases the offset.
When an object is not required, garbage collector reuses the object's memory for further allocation.
As objects forms tree, they have one or more root objects. If root objects are reachable, the whole tree is reachable. There are some special objects as well which are garbage collection roots (GC roots) and are always reachable.
